Many of the systems, we develop today needs some kind of a logging/tracing mechanism to identify issues or any kind of information.
NLog
NLog is a free logging framework to log various kind of messages into a specified place. We can use NLog in a .NET environment, as well as with Xamarin and Windows phone.
Logging
If we want to log a message from our application, We can use EventViewer without any libraries. But If we want to share the log file with someone else and analyse it in the future, we can’t do that with Eventviewer.
So we have to go for a logging framework if we want to write messages into a file or a database.
Logging Frameworks
We can use any of these frameworks for logging purpose, Log4net, NLog, ELMAH, Microsoft Enterprise Library, NSpring. ELMAH is a web logging framework, All the other frameworks can be used with any .NET type of application.
When we use NLog or Log4net for logging, it takes just few minutes to integrate it to the application, But performancewise its not the same. NLog is much faster than Log4net and as well as all the above mentioned logging frameworks.
NSpring also easy to setup, but it requires more coding to log a message. With a large scale enterprise application, It will be tedious to work with NSpring.
Microsoft Enterprise Library (EntLib) is much faster, But need heavy configurations and coding to work with it. ELMAH is a web logger, It’s going to log the messages into a xml file by default.
If you application needs file logging and more performance, better to go with NLog or NSpring. We can use Log4net also, it’s not that much faster, but easy to setup and use it in the application
Install NLog
Latest stable version of NLog is NLog 4.3.5.
With Nuget Package manager, I installed NLog,

NLog Targets
NLog targets are used to show, store or pass the message into another destination. Some commonly used NLog targets are shown below,
- Console : writes message to a console window.
- Event Log : writes message to event viewer.
- Debugger : writes messagee to the attatched debugger.
- File : writes log message to a file.
- Database : store log message in database.
- Memory : can write log message into a arraylist in memory.
- Mail : send log message by email.
- Method call : For log message, calls specific static method.
- Network : send log message over a network.
- Service : calls a web service for each log message.
And if these targets dont satisfy your requirements, you can create your own targets as well, (custom target)
Target Layout
Target layout means how our log message is going to display, in which format, which order. We can create custom layout renders as well. Commonly used layouts are as follows,
- ${callsite} : Class name, method name or source information of the log message.
- ${callsite-linenumber} : line no of call site source.
- ${date} : date and time
- ${exception} : exception details
- ${level} : log level
- ${logger} : logger name
- ${longdate} : date and time in long format.
- ${message} : log message.
- ${stacktrace} : stack trace
NLog rules
NLog rules are called as a log routing table. It’s going to matches with a target and writes into a log with specified layout. Some of the attributes in NLog rules,
- name : logger name,
- minlevel : minimum log level for a rule to match
- maxlevel : maximum log level for a rule to match
- level : single log level for a rule to match
- levels : comma seperated list of logs for a rule to match
- write to : comma seperated list of targets to be written when a rule matches
- final : no further rules are processed when this rule matches
- enabled : going to disable/enable rules
Nlog levels
One thing to note, In these level attribute, log messages are ordered from type. Trace is the minlevel log type, then Debug, Info, Warn, Error and Fatal.
- Trace level can be used when we need to notify the begining and end of a method
- Debug logs, we can identify whether session is expired, user is authenticated
- Info logs are used for more generic scenarios like, Email sent.
- Warn level can be used to notify warnings
- Error logs are used for exceptions and when application crashes.
- Fatal logs are highest level, used for most important cases.
Let’s see what’s in action
In configuration file, I have configured log type and log level, I have used file logging, Trace as a minimum logging level.

In code, I have written like this,
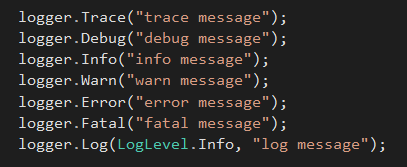
Let’s check how it’s written in the log file

In output, we can see the layout has been applied, ${longdate} ${callsite} ${callsite-linenumber} ${date} ${level} ${message}
How to use multiple targets
Let’s see how to add multiple targets for logging

In configuration file, i added two targets, one is to log into a file, other target is to log into a console window

File logging output is same as previous output, console output is seems like this. In console logging, I used a different layout like this, ${date} ${callsite} ${level} ${message} and minimmum log level is added as Warning level, So output got changed like this.
Some resources i referred,
I creted a github repo to these code samples, Please have a look, https://github.com/hansamaligamage/Logger